Make a delivery#
https://api.sandbox.harborlockers.com
https://api.harborlockers.com
Tip
Locker Statuses#
Before you reserve a locker, let’s go over what that means. It is essential for you, as a service provider, to understand what every locker status means. There are 6 possible statuses for a locker.
Available: The locker is available for any user to use.
Reserved: The locker is assigned to the creator of the reservation. Given this, no one can ask for a drop-off locker token except the reservation creator. If no drop-off locker token is asked, the locker will be available once the reservation expired (or you can explicitly cancel a reservation).
Rented: This happens when a user asks for a drop-off locker token. Now the locker cannot be accessed by anyone else.
Occupied: A drop-off open token was used. It is assigned to the user. Now the locker is ready for a pick up token.
Declined: The user has rented the locker but has cancelled their reservation. Can be changed by either using a release token or a drop off token.
Out of service: Not available to use and not assigned to anybody.
The following diagram explains the situtations that determine the locker’s state.
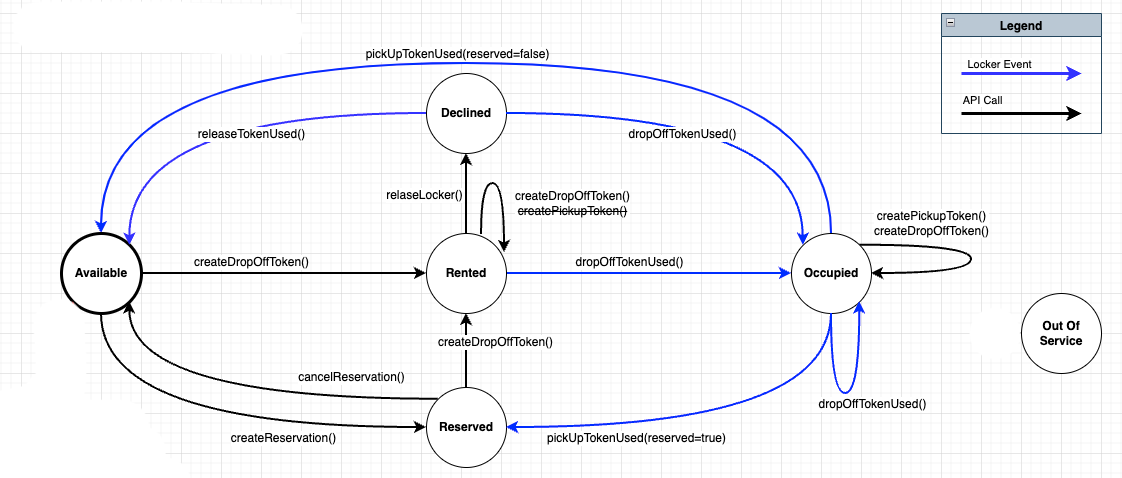
Now that you know what locker status means, let’s reserve a locker.
Finding lockers in your tower#
In order to find what lockers are in the tower:
curl -X 'GET' \
'{api_url}/api/v1/towers/{tower_id}/lockers' \
-H 'accept: application/json' \
-H 'Authorization: Bearer {access_token}'
Keep in mind this request will return ALL lockers and not just the available ones. So you will need some kind of filtering in your app to not show the unavailable ones to the cutomer.
[{"id":959,"name":"14","isLowLocker":false,"towerId":{tower_id},"status":{"name":"available","id":1},"type":{"name":"small","description":null,"id":1},"accessMode":"public"},
In order to access this locker you will need to use the first “id” and not the “name” In this case it is: 959.
Reserving a locker#
After finding the ID of the locker you want you can reserve the locker and give the user time to reach the location, let’s say 5 minutes.
# from the list, we select a locker_id and tower_id and make a reservation for 300 sec (5�min)
# Json request body => {'duration':300}. Number of seconds for the reservation
POST {api_url}/api/v1/towers/{tower_id}/lockers/{locker_id}/reservations
Now we want to give the user the key to open the locker. Here’s an example.
// requests encrypted tokens to open target locker
export async function createDropOffToken(towerId, lockerId, bearerToken) {
try {
const createDropOffEndpoint = `{api_url}${towerId}/lockers/${lockerId}/dropoff-locker-tokens`;
const requestOptionsAuth = {
headers: {
'Content-Type': 'application/json',
Accept: 'application/json',
Authorization: `Bearer ${access token}`,
},
};
const requestBody = {client_info: '', duration: 3000};
const response = await axiOS.post(
createDropOffEndpoint,
requestBody,
requestOptionsAuth,
);
return {
payload_auth: response.data.payload_auth,
payload: response.data.payload,
};
} catch (error) {
throw error;
}
}
Warning
Don’t exaggerate your token request, the locker will be in your possession until the token expires.
This function returns payload_auth
and payload
You will need these to open a reserved locker.
The locker opens and then the user puts the item inside and closes the locker.
Picking up the item#
Now the same or another user wants to pick up the item. Here are a couple of examples on how to do this.
Use the payload
and payload_auth
from creating the drop off token to open the locker.
HarborLockersSDK.sendOpenLockerWithTokenCommand(
payload,
payload_auth
);
func sendOpenLockerWithTokenCommand(_ payload: String, payloadAuth: String) throws {
guard let payloadAuthData = Data(hexString: payloadAuth),
let payloadData = Data(hexString: payload) else {
throw TestingError.init(.genericError, message: "Open locker failed")
}
HarborSDK.shared.sendOpenLockerWithToken(payload: payloadData, payloadAuth: payloadAuthData) { lockerId, error in
if let error = error {
print("Error sending token: \(error)")
} else {
print("Locker opened with ID: \(lockerId)")
}
}
}
fun sendOpenLockerWithTokenCommand(payload: String, payloadAuth: String) {
val payloadAuthData = payloadAuth.hexStringToByteArray()
val payloadData = payload.hexStringToByteArray()
if (payloadAuthData == null || payloadData == null) {
throw TestingError(GenericError, "Open locker failed")
}
HarborSDK.shared.sendOpenLockerWithToken(payload = payloadData, payloadAuth = payloadAuthData) { lockerId, error -> error?.let {
println("Error sending token: $error")
} ?: run {
println("Locker opened with ID: $lockerId")
}
}
}
The locker opens, the user removes the item and closes the locker.
Once the locker is closed, the locker will return to the Available state.
You can find information about debug logging and other tools to interact with lockers in SDK_tools
If anything has gone wrong in the test environment, you can use Harbor Console
If nothing has gone wrong: Congratulations! You have just made your first delivery!